起因
类似拥堵路段的绘制,我们需要在地图上根据风险程度,对不同的街道着以不同的颜色。
困难
关于绘制线段,Google Maps 只提供了 JavaScript API, 而我对JavaScript不熟。
数据文件在本地,需要进行预处理,JS并不擅长做数据处理和分析。
Google 提供的example只有相连的polyline(轨迹), 而我需要的是绘制很多割裂的线段。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no">
<meta charset="utf-8">
<title>Simple Polylines</title>
<style>
/* Always set the map height explicitly to define the size of the div
* element that contains the map. */
#map {
height: 100%;
}
/* Optional: Makes the sample page fill the window. */
html, body {
height: 100%;
margin: 0;
padding: 0;
}
</style>
</head>
<body>
<div id="map"></div>
<script>
// This example creates a 2-pixel-wide red polyline showing the path of
// the first trans-Pacific flight between Oakland, CA, and Brisbane,
// Australia which was made by Charles Kingsford Smith.
function initMap() {
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 3,
center: {lat: 0, lng: -180},
mapTypeId: 'terrain'
});
var flightPlanCoordinates = [
{lat: 37.772, lng: -122.214},
{lat: 21.291, lng: -157.821},
{lat: -18.142, lng: 178.431},
{lat: -27.467, lng: 153.027}
];
var flightPath = new google.maps.Polyline({
path: flightPlanCoordinates,
geodesic: true,
strokeColor: '#FF0000',
strokeOpacity: 1.0,
strokeWeight: 2
});
flightPath.setMap(map);
}
</script>
<script async defer
src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap">
</script>
</body>
</html>解决方案
最终我选择用Python进行数据处理,将得到的处理结果以字符串形式插入到HTML文本中,保存,即可在浏览器中查看结果。
注意: string.format() 插入参数处需用“{}”标识,原有的“{}”需用“{{}}”代替。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62def genHTML(filepath):
polylines, pathcolors = getDataFromFile(filepath)
return """
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no">
<meta charset="utf-8">
<title>Simple Polylines</title>
<style>
/* Always set the map height explicitly to define the size of the div
* element that contains the map. */
#map {{
height: 100%;
}}
/* Optional: Makes the sample page fill the window. */
html, body {{
height: 100%;
margin: 0;
padding: 0;
}}
</style>
</head>
<body>
<div id="map"></div>
<script>
// This example creates a 2-pixel-wide red polyline showing the path of
// the first trans-Pacific flight between Oakland, CA, and Brisbane,
// Australia which was made by Charles Kingsford Smith.
function initMap() {{
var map = new google.maps.Map(document.getElementById('map'), {{
zoom: 11,
center: {{lat: 40.65, lng: -74.00}},
mapTypeId: 'roadmap'
}});
var testpaths = [{pathdata}];
var testpathcolors = [{pathcolors}];
for (var i=0; i <testpaths.length; i++){{
var polyline = new google.maps.Polyline({{
path: testpaths[i],
geodesic: true,
strokeColor: testpathcolors[i],
strokeWeight: 2,
strokeOpacity: 1
}});
polyline.setMap(map);
}}
}}
</script>
<script async defer
src="https://maps.googleapis.com/maps/api/js?key={YOUR_API_KEY}&callback=initMap">
</script>
</body>
</html>
""".format(pathdata=polylines, pathcolors=pathcolors, YOUR_API_KEY="YourKey")
生成后的HTML文件中的关键片段, 其中分离的线段可以通过多次调用setMap()实现:
1 | <script> |
最终的结果:
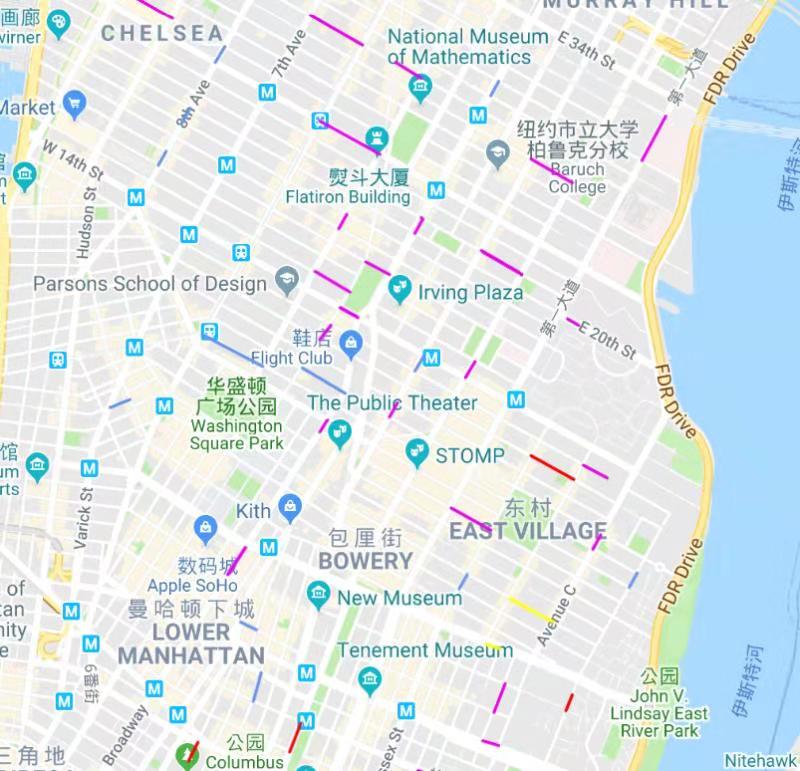